Colour by code in Excel with VBA - Part 2
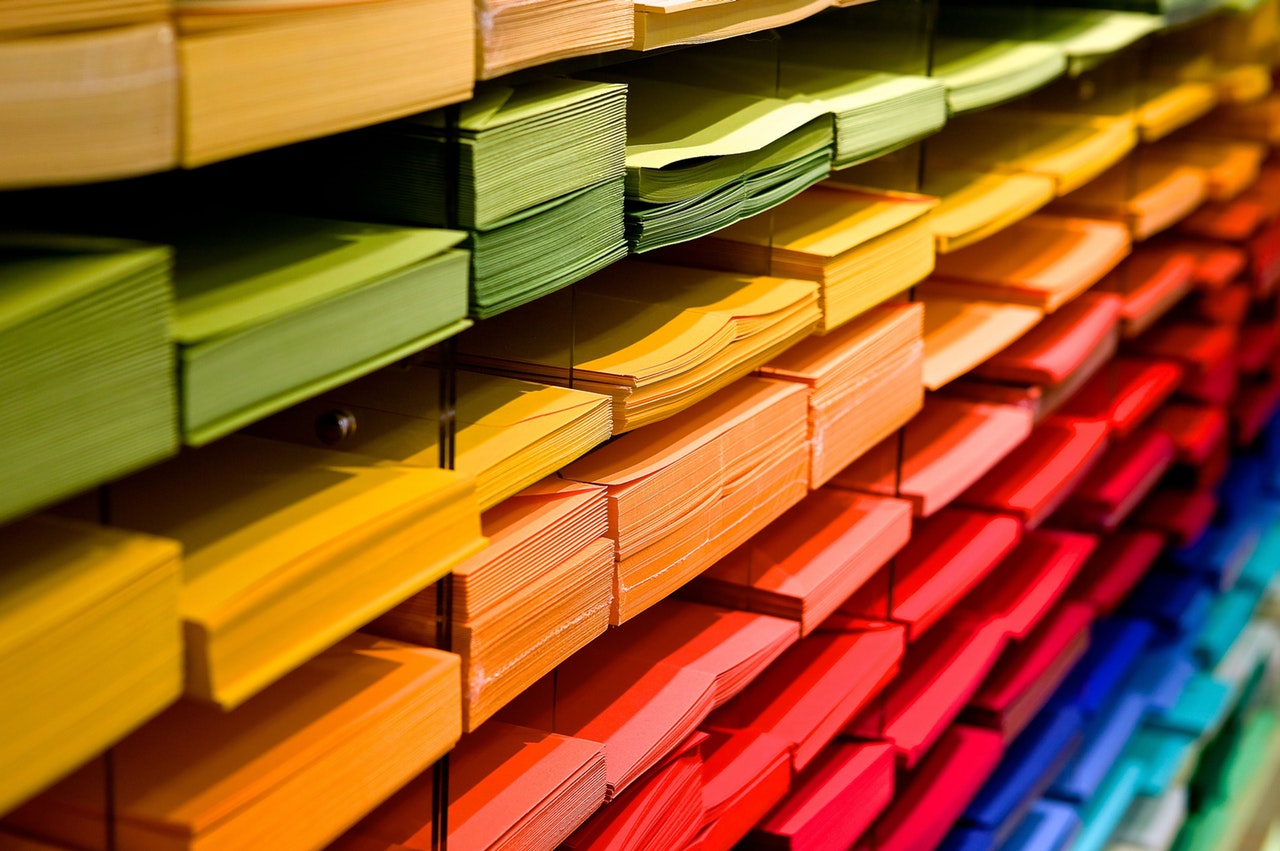
- 0 0
-
shares
Have you ever wanted to sample colours from a workbook to then use for other applications , like web pages, BI dashboards like QlikSense / OBIEE etc. , or even in Excel / VBA forms themselves?
Look no further, the following quick bit of code should help you achieve that with a very simple UDF that can return the right colour formatting for you with a copy/paste.
In recent years, Excel has added huge improvements to functionality in terms of sorting / filtering tables by colour, conditional formatting and palettisation of the tint/shades to be used with corporate themes.
There are some aspects of this that are inaccessible via the standard excel, namely - returning the hex colour value of a cell to use in HTML . For example, if your reporting is a hybrid of excel workbooks and common BI dashboards (Qliksense/ , Tableau / OBIEE etc. ) - with the code below, you'll be able to quickly mock up colours for charts in excel to use in your favourite BI tool, or simply just find the true RGB/HEX value of a particular custom colour in a sheet, to use in forms or across branding to keep themes consistent.
Here's the code
Pretty simple, takes in a range ( of one cell ) and returns , based on the parameter, the various colour codes to use in either web or other applications.
The function has an optional parameter, which returns a specific type or the excel generic colour number if left empty.
Colour | None | 1 | 2 | 3 | 4 | 5 | 6 | 7 | |
---|---|---|---|---|---|---|---|---|---|
Apples | 5287936 | 00B050 | 0, 176, 80 | 14 | &H0050B000& | 0 | 176 | 80 | |
Pears | 3506772 | 548235 | 84, 130, 53 | 12 | &H00358254& | 84 | 130 | 53 | |
Oranges | 4766194 | F2B948 | 242, 185, 72 | 44 | &H0048B9F2& | 242 | 185 | 72 | |
Peaches | 8892401 | F1AF87 | 241, 175, 135 | 40 | &H0087AFF1& | 241 | 175 | 135 | |
Bananas | 6740479 | FFD966 | 255, 217, 102 | 40 | &H0066D9FF& | 255 | 217 | 102 | |
Lemons | 65535 | FFFF00 | 255, 255, 0 | 6 | &H0000FFFF& | 255 | 255 | 0 | |
Cherries | 255 | FF0000 | 255, 0, 0 | 3 | &H000000FF& | 255 | 0 | 0 | |
Grapes | 10498160 | 7030A0 | 112, 48, 160 | 47 | &H00A03070& | 112 | 48 | 160 | |
Parameter values breakdown as below :
- Traditional HTML 'HEX' value
- Traditional comma separated Red,Green,Blue integer (0-255) values
- Traditional Excel colour index ( for the original palletised colours )
- The non-standard HEX format used in Excel / Office userforms for control colours
- Individual Red Integer component of 2 ( RGB )
- Individual Green Integer component of 2 ( RGB )
- Individual Blue Integer component of 2 ( RGB )
This should give you options to produce colour tables / outputs to then transfer into other applications, like QlikSense, OBIEE etc, and keep consistent colours across multiple formats.