Useful date functions
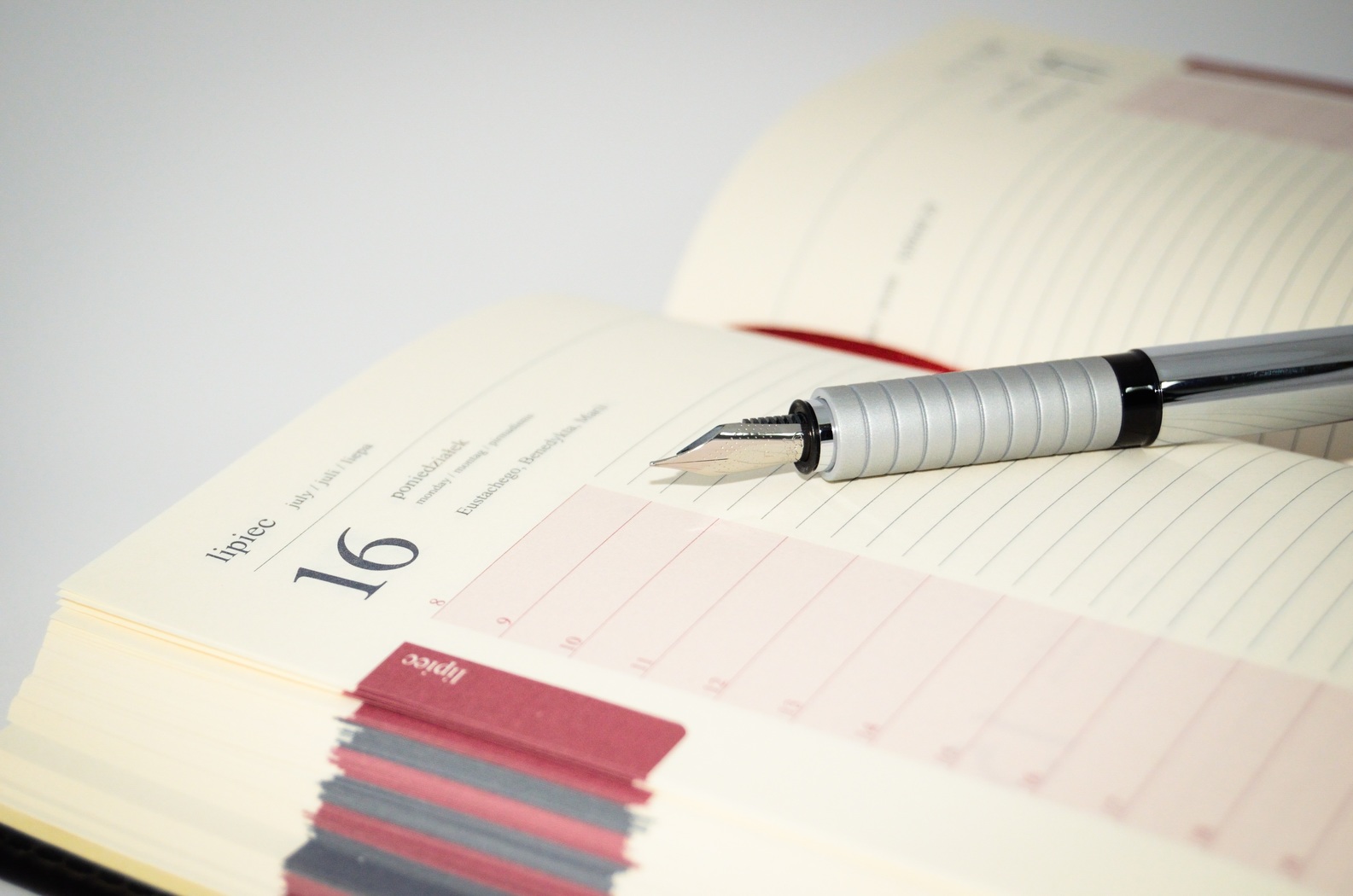
- 0 0
-
shares
Here are a selection of date functions I regularly use to either transform text into date values , or vice versa. Paste these into your project if you're presented with a sheet where users have manually typed such as '31st January 2012' or similar.
A quick rundown of what each does..
DateTextToValue ( Date String , String )
Does what it says on the tin, combining all other functions, will take dates in the format '31st January 2012' and turn them into an excel date format , in this case 40939.
This can then be read for measures/dimensions in pivot charts.
DateClearNth( Ordinal Number as String ) - returns Integer
This will take any ordinal number string i.e. '21st' or '3rd' and return an integer value.
DateMonTextNum ( MonthName as String ) - returns Integer ( limited to 1-12 )
This will take any month name as text i.e. "Jan" or "January" and return the corresponding month number.
OrdinalDate( Date , Output Type Integer ) - Returns String based on Type
Below is an example of the output :
- 0 - returns just the Ordinal Day portion only
- 1 - returns just the Ordinal Day + Month
- 3 - returns just the Ordinal Day + Month + Year
Finally in this set, I've included a function to split a string by delimiter, and return the numbered portion of the string.
SplitDel ( String , Delimiter, Index ) - returns string at Index
In the example above, the text string has spaces between components , so "2nd Feb 2017" can be broken down into :
SplitDel(B5," ",0 ) = "2nd"
SplitDel(B5," ",1 ) = "Feb"
SplitDel(B5," ",2 ) = "2017"